The Go Programming Language: A Deep Dive into its History, Philosophy, and Practice
Origins at Google (2007-2009)
In late 2007, in the depths of Google’s Mountain View campus, three distinguished engineers – Robert Griesemer, Rob Pike, and Ken Thompson – began sketching out a new programming language. Their goal was ambitious yet practical: create a language that would make software development at Google’s scale both more efficient and more enjoyable.
Rob Pike, known for his work on Unix at Bell Labs and the creation of UTF-8, brought his extensive systems programming experience. Ken Thompson, the principal inventor of Unix and recipient of the Turing Award, contributed his deep understanding of programming language design. Robert Griesemer, who had worked on Google’s V8 JavaScript engine, brought expertise in building fast, efficient compilation systems.
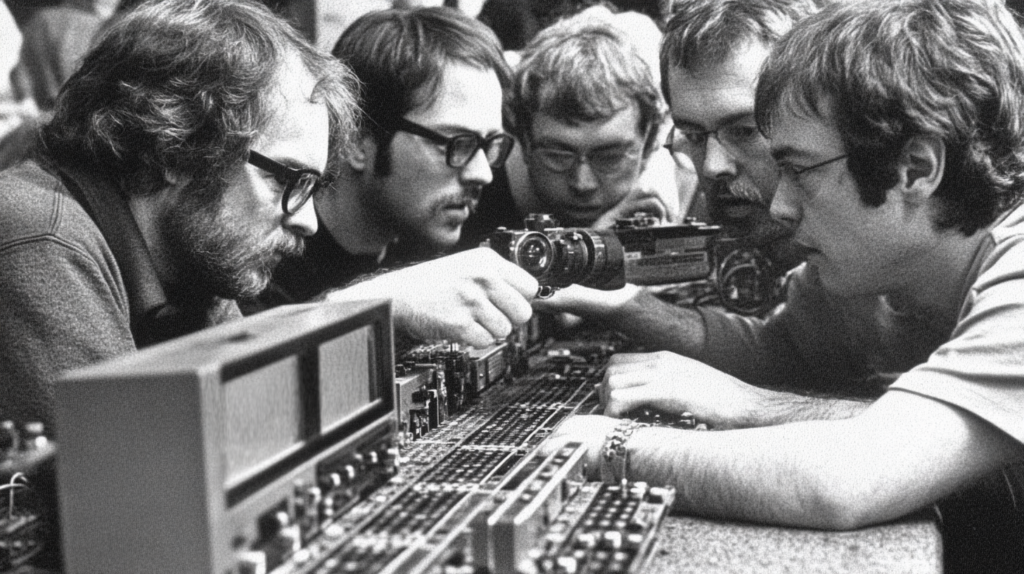
The trio was frustrated with the complexity of C++ and the long build times plaguing Google’s codebase. They envisioned a language that would combine:
- The efficiency of a compiled language
- The safety of a statically typed language
- The readability and ease of use of a dynamic language
- Built-in support for modern multicore processors
Core Philosophy
Go’s design philosophy can be summarized in three words: simplicity, readability, and practicality. As Pike famously stated: “Go is an attempt to combine the ease of programming of an interpreted, dynamically typed language with the efficiency and safety of a statically typed, compiled language.”
Here’s a simple example that demonstrates Go’s clarity:
package main
import (
"fmt"
"time"
)
func main() {
start := time.Now()
// Launch a goroutine
ch := make(chan string)
go func() {
time.Sleep(100 * time.Millisecond)
ch <- "Hello, concurrency!"
}()
// Wait for result
msg := <-ch
fmt.Printf("%s (%v elapsed)\n", msg, time.Since(start))
}
Key Innovations
1. Goroutines and Channels
Perhaps Go’s most significant contribution to mainstream programming is its approach to concurrency. Goroutines are lightweight threads managed by the Go runtime, allowing developers to spawn thousands or even millions of concurrent operations:
func fetchURLs(urls []string) []string {
results := make(chan string, len(urls))
for _, url := range urls {
go func(url string) {
// Simulated fetch
time.Sleep(100 * time.Millisecond)
results <- fmt.Sprintf("Result from %s", url)
}(url)
}
// Collect results
var responses []string
for i := 0; i < len(urls); i++ {
responses = append(responses, <-results)
}
return responses
}
2. Interface Satisfaction
Go’s interface system is uniquely powerful in its implicit satisfaction. Types implement interfaces simply by implementing their methods:
type Writer interface {
Write([]byte) (int, error)
}
type ConsoleWriter struct{}
// ConsoleWriter automatically satisfies Writer
func (c ConsoleWriter) Write(data []byte) (int, error) {
return fmt.Println(string(data))
}
3. Error Handling
Go’s error handling, while controversial, promotes explicit error checking and handling:
func readConfig(path string) (*Config, error) {
data, err := ioutil.ReadFile(path)
if err != nil {
return nil, fmt.Errorf("reading config: %w", err)
}
var config Config
if err := json.Unmarshal(data, &config); err != nil {
return nil, fmt.Errorf("parsing config: %w", err)
}
return &config, nil
}
The Evolution of Go
Go 1.0 (2012)
The first stable release established the language’s compatibility guarantee: code written for Go 1.0 would continue to compile and run correctly throughout all Go 1.x releases.
Go 1.5 (2015)
A milestone release where the compiler was rewritten in Go itself, eliminating the last C code from the toolchain. This release also introduced the concurrent garbage collector.
Go 1.11 (2018)
Introduced modules, revolutionizing Go’s dependency management:
module example.com/myproject
go 1.16
require (
github.com/gin-gonic/gin v1.7.4
github.com/go-sql-driver/mysql v1.6.0
)
Go 1.18 (2022)
Added generics, addressing one of the most requested features:
func Map[T, U any](s []T, f func(T) U) []U {
r := make([]U, len(s))
for i, v := range s {
r[i] = f(v)
}
return r
}
Modern Go Development
Today’s Go ecosystem is rich with tools and practices that make development efficient:
Testing
Go’s testing framework is built into the language:
func TestSum(t *testing.T) {
tests := []struct {
name string
numbers []int
expected int
}{
{"positive numbers", []int{1, 2, 3}, 6},
{"zero", []int{0}, 0},
{"empty slice", []int{}, 0},
}
for _, tt := range tests {
t.Run(tt.name, func(t *testing.T) {
if got := Sum(tt.numbers); got != tt.expected {
t.Errorf("Sum() = %v, want %v", got, tt.expected)
}
})
}
}
Performance Tools
Go includes powerful profiling tools:
import "runtime/pprof"
func main() {
f, _ := os.Create("cpu.prof")
pprof.StartCPUProfile(f)
defer pprof.StopCPUProfile()
// Your program here
}
Impact and Future
Go has significantly influenced modern software development, particularly in cloud infrastructure. Projects like Docker, Kubernetes, and HashiCorp’s suite of tools are written in Go, demonstrating its effectiveness for building complex distributed systems.
The language continues to evolve, with proposals for improved error handling, better generics support, and enhanced tooling. The Go team maintains its commitment to simplicity while carefully adding features that address real-world needs.
Conclusion
Go’s success stems from its careful balance of simplicity and power. Its focus on practical software engineering problems, combined with excellent tooling and a strong standard library, has made it a go-to language for modern backend development and cloud infrastructure.
As Rob Pike noted: “Go is not meant to innovate programming theory. It’s meant to innovate programming practice.” This philosophy has proven successful, as Go continues to gain adoption and influence the way we build software systems.